Unity Windows Phone Push Notification
Enabling push notification on Windows Phone platform while building on Unity is not straightforward specially if you are not from Windows Phone background. App42 makes this task seamless for unity developer and this can be done with minimal effort without prior knowledge of Windows platform.
This tutorial uses a sample app to give you a walkthrough of integration. Here are the few easy steps to get started with App42 Windows Phone Push Notification in Unity Applications.
1. Prerequisite Setup
- Register with App42 platform.
- Create an app once, you are on Quick start page after registration.
- If you are already registered, login to AppHQ console and create an app from App Manager -> App Create link.
- Go to the “Application Keys” and get Api key and Secret key.
2. Start building
- Download Unity sample project from our GitHub Repo
- Unzip the downloaded file and open project in Unity.
- Open Constants.cs file in Assets folder of sample project and pass required information.
- Change ApiKey and SecretKey that you have received in above step at line no 6 and 7.
- Change UserId with your user name you want to register for Push Notification at line no 8.
- Set the flag mNotificationType for type of pushNotification in OnGUI method of UseMyPlugin.cs.
- Add component UseMyPlugin.cs as script on main camera available in Assets folder.
UseMyPlugin.cs will do all the stuff for you seamlessly. Once app will get started it will register your app with MPNS as well as with App42 platform for push notification usage. It uses StoreDeviceToken method to register app with App42 server. This is only one time activity and will be done when app will be opened.
If you want to go in detail about the sample code, please see UseMyPlugin.cs file in Assets folder of sample project,in which user is registered on MPNS first and after getting Push notification Uri from MPNS, user registers on App42 platform to use Push Notification in Unity App. Callback.cs file is in Assets folder of sample project. Unity code snippet defined in UseMyPlugin.cs file of sample project is shown below
Initialization on Start-up
Following snippet of UseMyPlugin will do the initialization and calls for Registration with MPNS and App42 server on start up.
void Start()
{
m_ScreenRectangle = new Rect(0, 0, Screen.width, 100);
m_GUIStyle = new GUIStyle {fontSize = 16, alignment = TextAnchor.MiddleCenter,wordWrap=true};
#if UNITY_WP8
App42API.Initialize(Constants.ApiKey,Constants.SecretKey);
App42API.SetLoggedInUser(Constants.UserId);
App42PushService pushService=new App42PushService();
pushService.CreatePushChannel(Constants.UserId,true,PushChannelRegistrationCallback,PushChannelMessageCallback);
message="Push Channel Start";
#endif
}
Register with App42:
void PushChannelRegistrationCallback(object msg,bool IsError)
{
message=(string)msg;
if(!IsError)
{
StoreDeviceId((string)msg);
}
Debug.Log(message);
}
public void StoreDeviceId(string pushUri)
{
App42API.BuildPushNotificationService().StoreDeviceToken(App42API.GetLoggedInUser(),pushUri, Constants.deviceType,new Callback());
}
Get Toast Push Notification Message When device would get any Toast Push Message our dll will invoke PushChannelMessageCallback
void PushChannelMessageCallback(object sender,Dictionary<string,string> e)
{
// Parse out the information that was part of the message.
StringBuilder msg = new StringBuilder();
foreach (string key in e.Keys)
{
msg.AppendFormat("{0}: {1}\n", key, e[key]);
}
message=msg.ToString();
Debug.Log(message);
}
- Build your library project.
- After Building Windows Phone Project from Unity, We will have to Modify WMAppManifest file to
Add capabilities. Copy the below code in WMAppManifest file to capabilities section when you are integrating with existing project.
<Capability Name="ID_CAP_PUSH_NOTIFICATION" />
<Capability Name="ID_CAP_PROXIMITY" />
3. Integration and Customization in Your Existing Project.
If you are integrating in your existing Unity Windows Phone application for Push Notification,Do follow the below steps
4. Sending Message to User
Using Unity Code
Once your app is registered you are ready to send message to your app user using following code snippet from any App42 Unity SDK . Since we are supporting two types of push messages toast message and tile message so there is two methods for them.To send Tile push message we create Tile class object and set the parameters.
For “backBackgroundImage” and “backgroundImage” parameter either we can set any local image or any web image URI.
public void sendPushToUser(string userName,string msg){
switch (mNotificationType)
{
case NotificationType.TOAST:
//To send Toast Push Message
App42API.BuildPushNotificationService().SendPushMessageToUser(userName, "msg", new Callback()); break;
case NotificationType.TILE:
Tile tile = new Tile();
tile.title = "Tile";
tile.backTitle = "Tile Message";
tile.count = "10";
tile.backContent = "back";
tile.backBackgroundImage = "Blue.jpg";
tile.backgroundImage = "Red.jpg";
// To send Tile Push message
App42API.BuildPushNotificationService().SendPushTileMessageToUser(userName, tile, new Callback());
break;
}
}
Using AppHQ Console :
You can also send push messages to your registered app user directly from AppHQ console. To do this follow the simple steps:
- Login to AppHQ Management Console, Click on Unified Notification
- Click on Push -> Click on Users
- Select your App and user
- Click on Manage from table and click on send push button as shown in the below image:
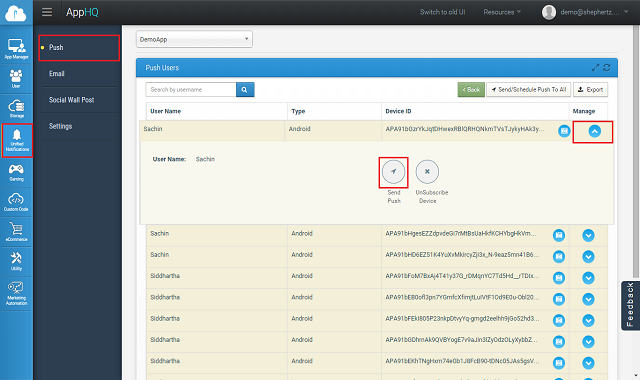
Type your message, once you are done click on send button as show below. This will trigger a push notification message to your app user
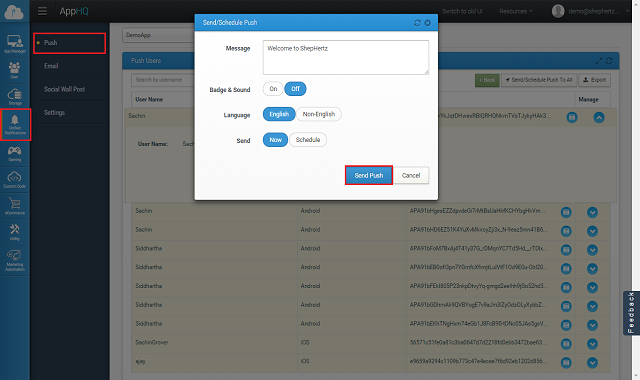
5. Sending Message to iOS Device with Sound and Badge
If you want to send push message with sound and badge effect on iOS device, you have to pass JSON string in below format.
string userName = "Nick";
string message = "{'alert':'Hi There...','badge':1,'sound':'default'}";
App42API.BuildPushNotificationService().SendPushMessageToUser(userName ,message ,new Callback());
You can also do it from AppHQ by putting this JSON format in message text area for sending message to iOS device with sound and badge effect.
6. Sending Message to Channel
App42 Push notification also supports channel subscription model where user can subscribe on channel of his interest to receive the notification. You can send message to channel which will deliver message to all users who are subscribed to that channel.
A channel can be created from AppHQ console. To do this follow the simple steps:
- Login to AppHQ Management Console, Click on Unified Notification
- Click on Push -> Click on Channels
- Select your App -> Click on Add channel button
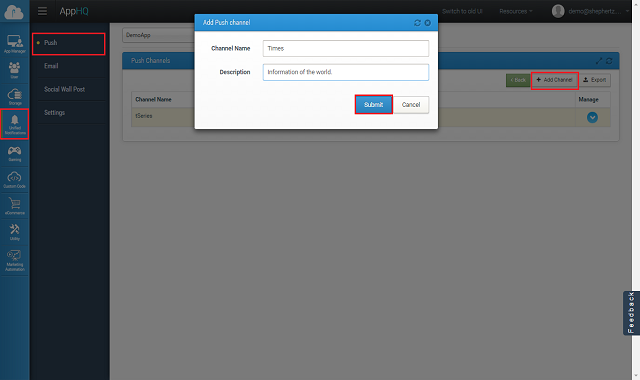
Once channel is created you can ask user for subscription on the channel. Below is the code snippet for the same
Unity Method :
Here is Unity Code to send Push Notification to Channel.
string channelName = "cricket channel";
string description= "channel description";
//user name must be registered already for Push Notification
string userName = "Nick";
//Create Channel
PushNotificationService pushNotificationService =App42API.BuildPushNotificationService();
pushNotificationService.CreateChannelForApp (channelName , description,new Callback());
//Subscribe user to that channel
pushNotificationService .SubscribeToChannel (channelName ,userName,new Callback());
// Now send message on the Channel
string message = "Mumbai Indians won IPL 6";
PushNotification pushNotification = pushNotificationService.SendPushMessageToChannel(channelName ,message,new Callback());
7. Scheduling Message to User
You can also schedule message to your app user on specified time from AppHQ console. To do this follow the simple steps:
- Login to AppHQ Management Console, Click on Unified Notification
- Click on Push -> Click on Users
- Select your App -> select target user and click on manage
- Click on Send button and type your message in pop up and pass your time to schedule the message.
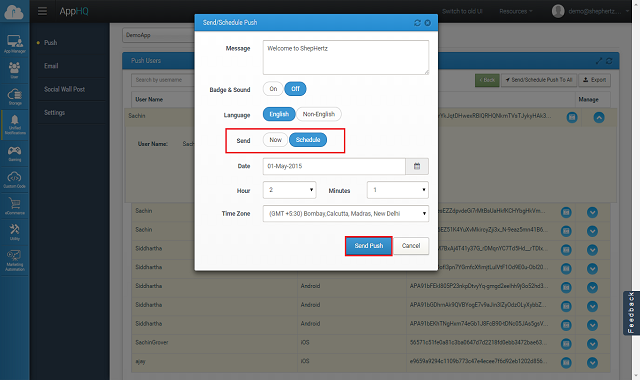
8. Scheduling message to Channel
Message scheduling on channel can be done in similar way. To do this follow the simple steps:
- Login to AppHQ Management Console, Click on Unified Notification
- Click on Push -> Click on Channels
- Select your App -> select target channel and click on manage
- Click on Send Push button and type your message in pop up and pass your time to schedule the message.
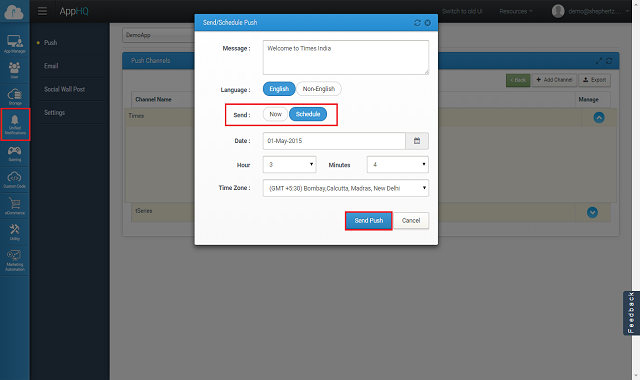
9. Doing Push Analytics
Push notification goes from App42 server to MPNS server and then device. Delivery of Push notification is not guaranteed from MPNS to device and it is stated as best effort. Once it is delivered on user device, there might be a chance that user clears it without actually Opening the message.
Using App42 Push Analytics, you can track how many push notification was sent from your side, how many were delivered and how many were opened by the user.
You can see these analytics from AppHQ console which will give a better insight of push notification campaign.
If you want to track push message read/opened event you have to simply put following snippet after Message is clicked and lands to your activity. This will enable tracking of push message and analytics can be seen from AppHQ console.
App42API.BuildLogService().SetEvent("Message", "Opened", new Callback());
10. Send Multilingual Push Notification
Once your device is registered for Push Notification you are ready to send multilingual(UTF-8) push message to your app user using following code snippet from any App42 SDK (Android/Java/WP etc)
String userName = "Nick";
String message = "Message which you have to send";
App42Log.SetDebug(true); //Print output in your editor console
Dictionary<String, String> otherMetaHeaders = new Dictionary<String, String>();
otherMetaHeaders.Add("dataEncoding", "true");
pushNotificationService.SetOtherMetaHeaders(otherMetaHeaders);
pushNotificationService.SendPushMessageToUser(userName,message, new UnityCallBack());
public class UnityCallBack : App42CallBack
{
public void OnSuccess(object response)
{
PushNotification pushNotification = (PushNotification) response;
App42Log.Console("Message is " + pushNotification.GetMessage());
App42Log.Console("userName is : " + pushNotification.GetMessage());
}
public void OnException(Exception e)
{
App42Log.Console("Exception : " + e);
}
}
For more details of Push Notification Documentation